Use the docker stop command to stop a container: docker stop option containerid. Replace containerid with the container’s name or ID. By default, you get a 10 second grace period. The stop command instructs the container to stop services after that period. Use the -time option to define a different grace period expressed in seconds. And I did not think about docker pause and docker unpause, which might solve the stop-start pb. Gotta rush right now, but if volumerise could look for containers that would include the string yourprojectnameyourcontainername. and pause-unpause them might solve the issue.
-->In this tutorial, you'll learn about creating and deploying Docker apps, including using multiple containers with a database, and using Docker Compose. You'll also deploy your containerized app to Azure.
Start the tutorial
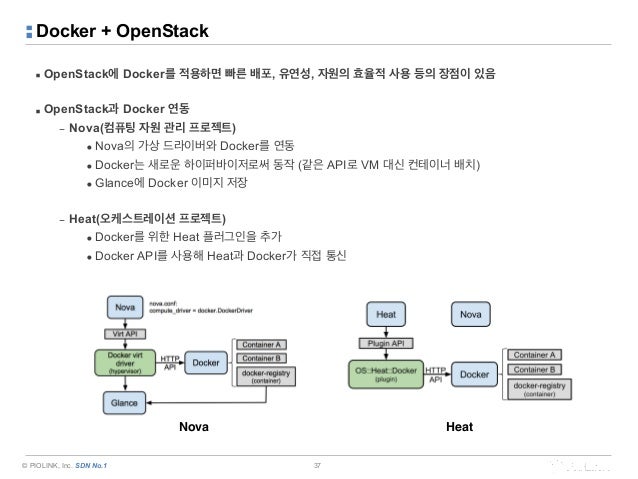
If you've already run the command to get started with the tutorial, congratulations! If not, open a command prompt or bash window, and run the command:
You'll notice a few flags being used. Here's some more info on them:
-d
- run the container in detached mode (in the background)-p 80:80
- map port 80 of the host to port 80 in the containerdocker/getting-started
- the image to use
Tip
You can combine single character flags to shorten the full command.As an example, the command above could be written as:
The VS Code Extension
Before going too far, we want to highlight the Docker VS Code Extension, which gives you a quick view of the containers running on your machine. It gives you quickaccess to container logs, lets you get a shell inside the container, and lets you easily manage container lifecycle (stop, remove, and so on).
To access the extension, follow the instructions here. Use the Docker icon on the left to open the Docker view. If you open the extension now, you will see this tutorial running! The container name (angry_taussig
below) is a randomly created name. So, you'll most likely have a different name.
What is a container
Now that you've run a container, what is a container? Simply put, a container is simply another process on your machine that has been isolated from all other processes on the host machine. That isolation leverages kernel namespaces and cgroups, features that have been in Linux for a long time. Docker has worked to make these capabilities approachable and easy to use.
Note
Creating Containers from ScratchIf you'd like to see how containers are built from scratch, Liz Rice from Aqua Security has a video in which she creates a container from scratch in Go:
What is a container image
When running a container, it uses an isolated filesystem. This custom filesystem is provided by a container image. Since the image contains the container's filesystem, it must contain everything needed to run an application - all dependencies, configuration, scripts, binaries, and so on. The image also contains other configuration for the container, such as environment variables, a default command to run, and other metadata.
We'll dive deeper into images later on, covering topics such as layering, best practices, and more.
Note
If you're familiar with chroot
, think of a container as an extended version of chroot
. The filesystem is simply coming from the image. But, a container adds additional isolation not available when simply using chroot.
Next steps
Continue with the tutorial!
Estimated reading time: 4 minutes
A container’s main running process is the ENTRYPOINT
and/or CMD
at theend of the Dockerfile
. It is generally recommended that you separate areas ofconcern by using one service per container. That service may fork into multipleprocesses (for example, Apache web server starts multiple worker processes).It’s ok to have multiple processes, but to get the most benefit out of Docker,avoid one container being responsible for multiple aspects of your overallapplication. You can connect multiple containers using user-defined networks andshared volumes.
The container’s main process is responsible for managing all processes that itstarts. In some cases, the main process isn’t well-designed, and doesn’t handle“reaping” (stopping) child processes gracefully when the container exits. Ifyour process falls into this category, you can use the --init
option when yourun the container. The --init
flag inserts a tiny init-process into thecontainer as the main process, and handles reaping of all processes when thecontainer exits. Handling such processes this way is superior to using afull-fledged init process such as sysvinit
, upstart
, or systemd
to handleprocess lifecycle within your container.
If you need to run more than one service within a container, you can accomplishthis in a few different ways.
Put all of your commands in a wrapper script, complete with testing anddebugging information. Run the wrapper script as your
CMD
. This is a verynaive example. First, the wrapper script:Next, the Dockerfile:
If you have one main process that needs to start first and stay running butyou temporarily need to run some other processes (perhaps to interact withthe main process) then you can use bash’s job control to facilitate that.First, the wrapper script:
Use a process manager like
supervisord
. This is a moderately heavy-weightapproach that requires you to packagesupervisord
and its configuration inyour image (or base your image on one that includessupervisord
), along withthe different applications it manages. Then you startsupervisord
, whichmanages your processes for you. Here is an example Dockerfile using thisapproach, that assumes the pre-writtensupervisord.conf
,my_first_process
,andmy_second_process
files all exist in the same directory as yourDockerfile.
Docker Start Container Docker For Macpicturelasopa Top
docker, supervisor, process management